To use WP REST API from another website to display WooCommerce products, we first need to generate new API keys under WooCommerce > Advanced > REST API
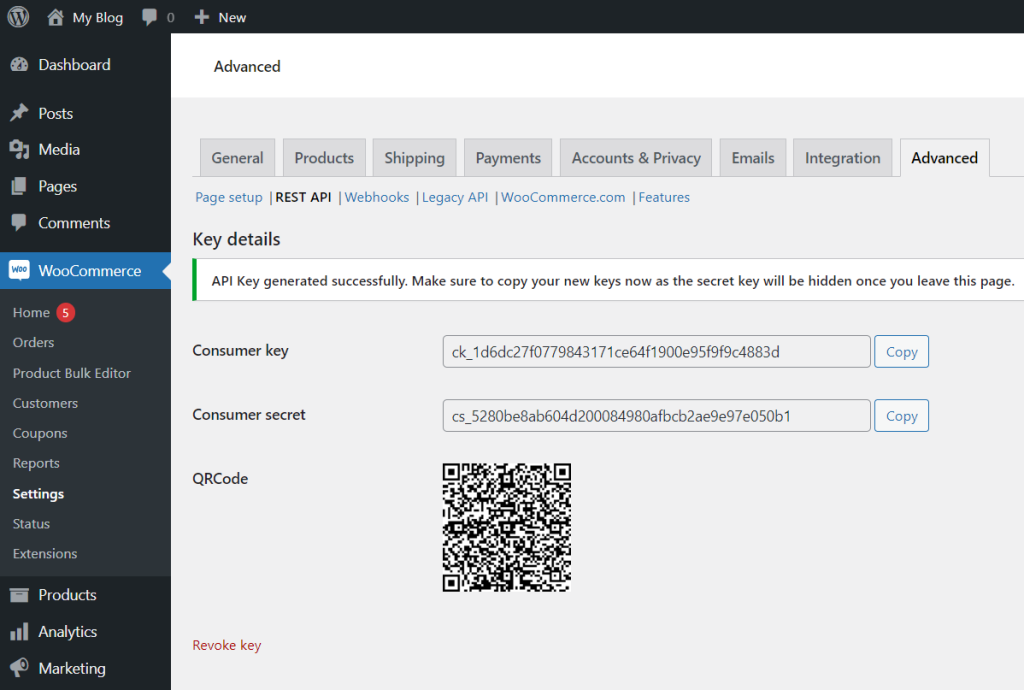
We can then use these keys along with the website URL to display the data:
<?php
$website_url = "https://plugins.club";
$consumer_key = "ck_1d6dc27f0779843171ce64f1900e95f9f9c4883d";
$consumer_secret = "cs_5280be8ab604d200084980afbcb2ae9e97e050b1";
$product_request_url = $website_url . '/wp-json/wc/v3/products';
$product_args = array(
'headers' => array(
'Authorization' => 'Basic ' . base64_encode( $consumer_key . ':' . $consumer_secret ),
),
);
$product_response = wp_remote_get( $product_request_url, $product_args );
$product_body = wp_remote_retrieve_body( $product_response );
echo $product_body;
💡 Make sure to replace website_url, consumer_key and consumer_secret with your data.
By default, WP REST API retrieves 10 products, but we can increase it up to 100 by adding ?per_page=100 to the url.
$product_request_url = $website_url . '/wp-json/wc/v3/products?per_page=100';
The data is output as json:
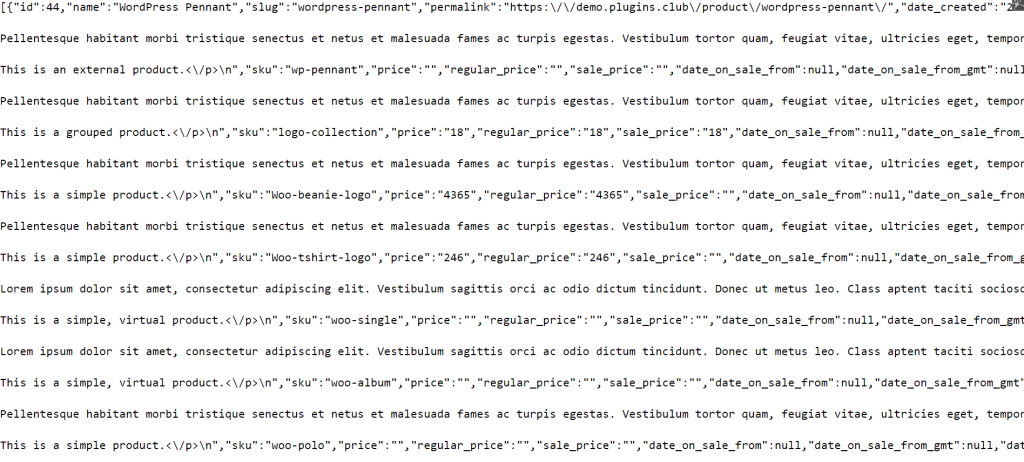
In case there are problems with the api, let’s add a check that will display the error:
$product_response = wp_remote_get( $product_request_url, $product_args );
if ( is_wp_error( $product_response ) ) {
$error_message = $product_response->get_error_message();
echo "Something went wrong: $error_message";
} else {
$product_body = wp_remote_retrieve_body( $product_response );
echo $product_body ;
}
For example, if api keys are incorrect or missing, we will get an error:

moving on, let’s decode the JSON and put it in array $product_list:
} else {
$product_body = wp_remote_retrieve_body( $product_response );
$product_list = json_decode( $product_body );
}
so that we can display only what we want, and style it as a table:
} else {
$product_body = wp_remote_retrieve_body( $product_response );
$product_list = json_decode( $product_body );
$table = '<table><thead><tr><th>ID</th><th>Image</th><th>Name</th><th>Type</th><th>Slug</th><th>SKU</th><th>Price</th><th>Sale Price</th><th>Variations</th><th>Stock</th><th>Quantity</th><th>Category</th><th>Tags</th></tr></thead><tbody>';
foreach ( $product_list as $product ) {
$id = $product->id;
$image = $product->images[0]->src;
$name = $product->name;
$type = $product->type;
$slug = $product->slug;
$sku = $product->sku;
$price = $product->price;
$sale_price = $product->sale_price;
$variations = count($product->variations);
$stock = $product->stock_quantity;
$quantity = $product->quantity;
$category = $product->categories[0]->name;
$tags = implode(", ", array_map(function($tag) { return $tag->name; }, $product->tags));
$table .= "<tr><td>$id</td><td><img src='$image' alt='$name' style='max-width: 50px; max-height: 50px;'></td><td>$name</td><td>$type</td><td>$slug</td><td>$sku</td><td>$price</td><td>$sale_price</td><td>$variations</td><td>$stock</td><td>$quantity</td><td>$category</td><td>$tags</td></tr>";
}
$table .= '</tbody></table>';
echo $table;
}
Result:
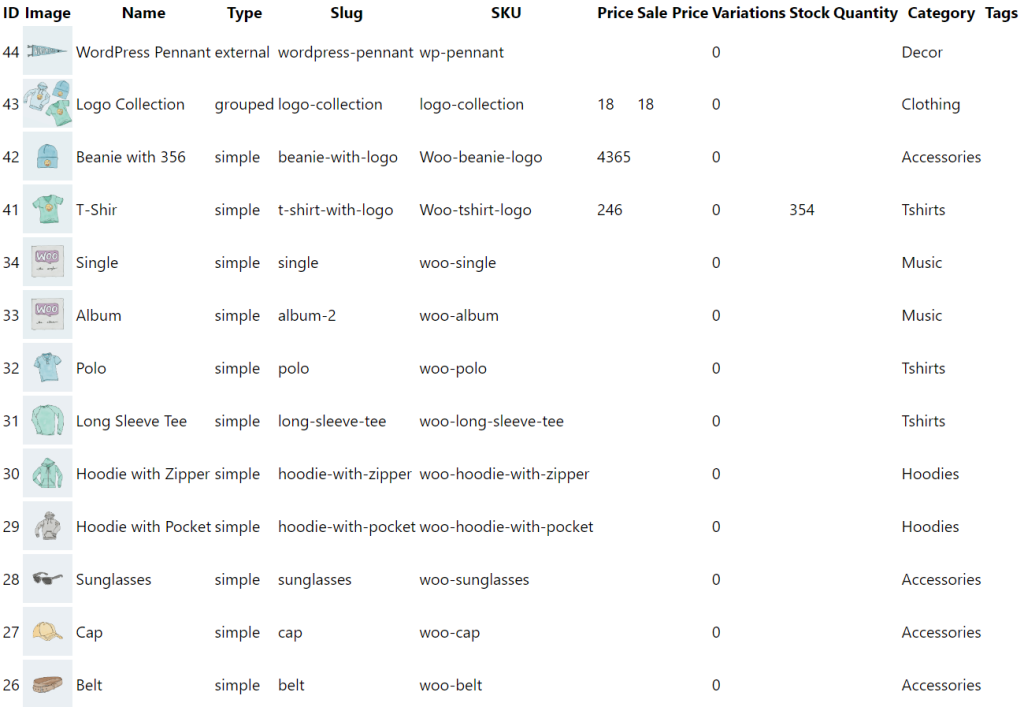
Final code:
<?php
$website_url = "https://plugins.club";
$consumer_key = "ck_1d6dc27f0779843171ce64f1900e95f9f9c4883d";
$consumer_secret = "cs_5280be8ab604d200084980afbcb2ae9e97e050b1";
$product_request_url = $website_url . "/wp-json/wc/v3/products?per_page=100";
$product_args = [
"headers" => [
"Authorization" =>
"Basic " . base64_encode($consumer_key . ":" . $consumer_secret),
],
];
$product_response = wp_remote_get($product_request_url, $product_args);
if (is_wp_error($product_response)) {
$error_message = $product_response->get_error_message();
echo "Something went wrong: $error_message";
} else {
$product_body = wp_remote_retrieve_body($product_response);
$product_list = json_decode($product_body);
$table =
"<table><thead><tr><th>ID</th><th>Image</th><th>Name</th><th>Type</th><th>Slug</th><th>SKU</th><th>Price</th><th>Sale Price</th><th>Variations</th><th>Stock</th><th>Quantity</th><th>Category</th><th>Tags</th></tr></thead><tbody>";
foreach ($product_list as $product) {
$id = $product->id;
$image = $product->images[0]->src;
$name = $product->name;
$type = $product->type;
$slug = $product->slug;
$sku = $product->sku;
$price = $product->price;
$sale_price = $product->sale_price;
$variations = count($product->variations);
$stock = $product->stock_quantity;
$quantity = $product->quantity;
$category = $product->categories[0]->name;
$tags = implode(
", ",
array_map(function ($tag) {
return $tag->name;
}, $product->tags)
);
$table .= "<tr><td>$id</td><td><img src='$image' alt='$name' style='max-width: 50px; max-height: 50px;'></td><td>$name</td><td>$type</td><td>$slug</td><td>$sku</td><td>$price</td><td>$sale_price</td><td>$variations</td><td>$stock</td><td>$quantity</td><td>$category</td><td>$tags</td></tr>";
}
$table .= "</tbody></table>";
echo $table;
}
We can now further modify the data to style it better, or even use it to create affiliate WooCommerce products on our own store using product data from this other store.